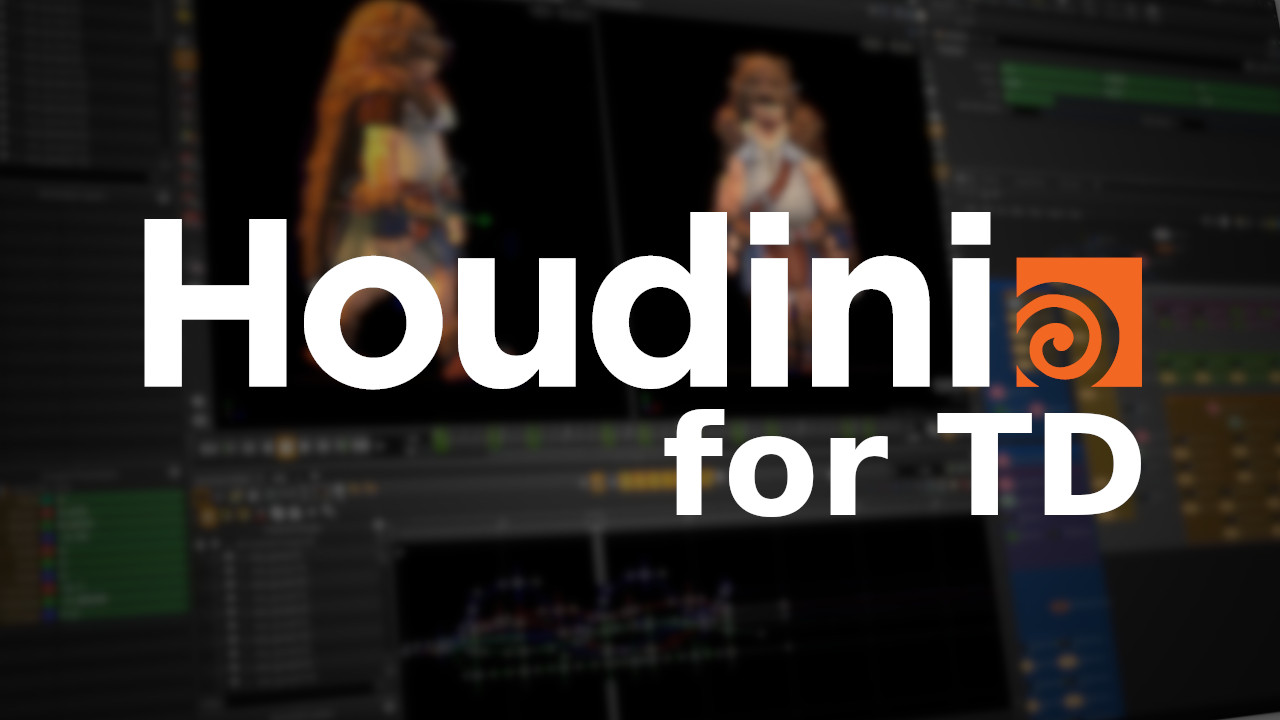
Note
This article is part of the serie Houdini for TD
Creating custom ROPs
If you are looking to implement a new renderer, using the regular HDA mechanic won't be working, as it's mechanic would be restraint to only create a collection of ROP nodes to be executed.
In order to create a new Renderer, you will have to create a new asset by going in the main menu and select File > New Asset... then select Output Driver as Definition.
Important
If your HDA is not a Output Driver, calling `hou.RopNode.render()` won't trigger any action.
SOHO
Once you have created a new Output Driver, pressing the render button won't stil do much. You will have to create the following parameters on your node:
Parm Name | Type | Usage |
---|---|---|
soho_program | String | The python script to run. |
soho_outputmode | Integer |
What to do with the printed output of the script. 0 is sent to soho_pipecmd, 1 to soho_diskfile, 2 goes to standard stdout. |
soho_pipecmd | String | The program that printed output is piped in. |
soho_diskfile | String | The file on disk to redirect printed output to. |
The soho program script will be able to import the soho module provided by SideFX, which allow your script to get information from your houdini ROP node.
Here is a quick example on how to get various kind of parameters values:
# my_render_script.py
import soho
# This is how you can get attributes value
int_value = soho.getDefaultedInt("my_int_parm", [1])[0]
float_value = soho.getDefaultedFloat("my_float_parm", [1.234])[0]
string_value = soho.getDefaultedString("my_string_parm", ['default_value'])[0]
# You can also get all the values from a parmTuple
# Here is to get the framerange from the node
start, end, inc = soho.getDefaultedInt("f", [1, 24, 1])
# To get the current render evaluation time
now = soho.getDefaultedFloat("state:time", [0.0])[0]
# Once you have all your parameters value, you can you what you want
# even interacting with the UI
if hou.isUIAvailable():
hou.ui.displayMessage("Hello World")
else:
print("Hello World")
Note
From houdini, it is possible to customize the output file via a render argument:
`hou.RopNode.render(output_file="/path/output.file")`
You can access this value in your soho program via:
`soho.getDefaultedString("vm_picture", [""])[0]`
For more details, please visit the SOHO documentation.
Customize Render Submission
When you call `hou.RopNode.render()`, houdini will invoke the render process on each node that are connected above to the rendered RopNode.
If you want to customize the submission, the easiest way is to create an extra button that has as a callback the submission function you want to use. You then can use the `hou.RopNode.inputDependencies()` method to fetch all the other inputs ROPs that need to be rendered.
Tasks Operations & PDG
PDG or TOPs (Tasks OPerations) can be used to manipulate results of the work by building network of TOPs nodes.
As it is quite complex, I will let your browse threw the PDG Documentation to learn how to use it.
Though I will still go threw some of the advantages and disadvantages that I found to such system. And before we start, here are 4 important PDG concept:
- WorkItems - a tasks to execute
- Processors - an operator used to generate WorkItems (tasks to execute)
- Partitions - an operator used to customize relationship between WorkItems
- Scheduler - Responsible to dispatch and execute WorkItems commands
Work with any Scheduler
PDG is not responsible to dispatch tasks to workstation on the farm but simply to generate the job and tasks that need to be executed. It delegate this to existing scheduler like Deadline, Tractor or HQueue, while also giving you the ability to adapt it to your own.
Dynamic Tasks Dependency
Tasks will be dispatched to the scheduler as they are finished allowing faster processing. Additionally, WorkItems have the ability to carry forward information to dependent WorkItems via the intermediates of Attributes, which can be used to customize downstream tasks.
Forwarding Data over Tasks
TOPs goes a steps farther than ROPs in visualizing dependency as in addition to see relationship between each Processors, you can also see the full dependency between WorkItems. Before I forget, one of the particularity and strengh of PDG, is the ability to generate tasks on the fly, as tasks finish.
Pricing and Integration
One of the major downside of PDG is that you need to pay for it.
Sometime writting simple python is much easier.